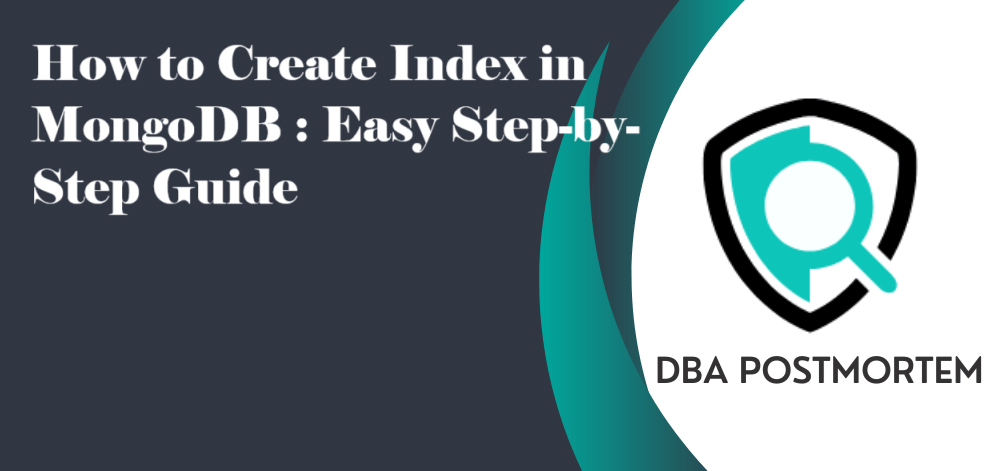
Index in MongoDB has a significant role to improve query performance by allowing efficient data retrieval. If the index is not there, MongoDB used to scan every document in a collection, which leads to slow queries. This article will walk you through how to create index in MongoDB. It will explain different types of indexes in MongoDB and provide how to optimize performance by creating indexes.
Table of Contents
1. Importance of Index in MongoDB :
If the proper index is not there, MongoDB performs a collection scan checking each document in a collection to find the required data which is obviously time-consuming. With indexing, the database can quickly search the relevant documents, improving query performance. Here are its benefits of it:
- Faster query execution
- Reduces unnecessary Resource Consumption (CPU and memory usage etc)
- Enhances sorting and filtering performance
2. Types of Indexes in MongoDB :
There are various types of Indexes like Single Field Index, Compund Index, Unnique Index, Sparse Index, Text Index etc. Let’s go through this with examples :
3. How to Create Index in MongoDB :
1. Single Field Index :
It will be created in a single field as below :
Syntax :
db.collection.createIndex({ field: 1 }) // 1 for ascending order, -1 for descending
Example :
db.employee.createIndex({ name: 1 }) // Creates an ascending index on the 'name' field
2. Compund Index(Multiple Field Index) :
It will be created on multiple fields as below :
Syntax :
db.collection.createIndex({ field1: 1, field2: -1 })
Example :
db.employee.createIndex({ name: 1, city: -1 })
It creates an ascending index on name
and a descending index on city
.
3. Unique Index :
We have to ensure that the collection does not contain duplicate values before creating a unique index as duplicate values cannot exist for the indexed field in an Unique index.
Syntax :
db.collection.createIndex({ field: 1 }, { unique: true })
Example :
db.employee.createIndex({ phno: 1 }, { unique: true })
4. Partial Index :
Partial index indexes only documents which matche a specified filter criteria/condition.
db.employee.createIndex({ status: 1 }, { partialFilterExpression: { status: { $exists: true } } })
Only documents where status
exists will be indexed. Other filters will not be considered.
5. Sparse Index :
This is an index which only includes documents where the indexed field exists. Documents without the indexed field are not included in the index. Let’s understand with an example.
Example : Consider an employee collection where some documents have a phone
field and others do not.
db.employee.insertMany([
{ name: "Ram", phone: "1234567890" },
{ name: "John" }, // No phone field
{ name: "Harish", phone: "9876543210" }
])
Now, let’s create a sparse index on the phone
field:
db.employee.createIndex({ phone: 1 }, { sparse: true })
Now, this will use the sparse index:
db.employee.find({ phone: "9876543210" }).explain("executionStats")
But below query will perform a collection scan, it won’t use the index because this includes missing fields as phone is null.
db.employee.find({ phone: null }).explain("executionStats")
6. Text Index :
A Text Index is a special type of index in MongoDB which enables efficient text search on string fields within the documents. It allows you to search for words, phrases, or patterns in a case-insensitive manner.
Syntax :
db.collection.createIndex({ field_name: "text" })
A text index can be created on one or multiple string fields. Let’s say we have a Countries collection with documents like:
{ "_id": 1, "name": "USA", "description": "Europian Country" }
{ "_id": 2, "name": "India", "description": "Multiple Cultures are there" }
{ "_id": 3, "name": "UAE", "description": "Futuristic Country" }
Now, create a Text Index on the text field:
db.countries.createIndex({ content: "text" })
The { content: "text" }
part tells MongoDB to create a text index on the content
field.
Now, perform a text search :
Example 1: Searching for “culture”
db.countries.find({ $text: { $search: "culture" } })
Output :
{ "_id": 2, "name": "India", "description": "Multiple Cultures are there" }
Example 2: Searching for Multiple Words
db.countries.find({ $text: { $search: "Europian Cultures" } })
This will return both the USA and India documents because they match either “Europian” or “Cultures“.
Output :
{ "_id": 1, "name": "USA", "description": "Europian Country" }
{ "_id": 2, "name": "India", "description": "Multiple Cultures are there" }
So, here are the detailed example of MongoDB create index and type of indexes in MongoDB as well. Hope this helps! Also, you can get more detailed information and more types of indexes in MongoDB from the Official Doc as well. This will explain the Use Cases as well.
Other MongoDB Related Articles :